Our goal is to build a simple and lightweight set of tools to generate, analyze, and process Java code.
All of this is offered under a business friendly license.
JavaParser is used in dozens of open-source and commercial projects. Show your love adding one star more or spreading the word.
What you can do with JavaParser
JavaParser is a set of tools to:
Java code.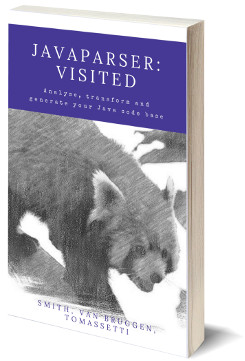
Want to learn JavaParser?
We are writing a book on JavaParser and we need your feedback!
Help us understanding what is not clear, what should we add, share examples and ideas with us. You can also contact us on gitter or on the book repository.
It would be great if you could help us spread the word:
Tweet!In the rest of the page we will look on different features of JavaParser. You may also be interested in checking out some presentations we gave about JavaParser.
Parsing Java code
Using JavaParser you can get an Abstract Syntax Tree (AST) from Java code. The AST is a structure representing your Java code in a way that it is easy to process:
CompilationUnit compilationUnit = JavaParser.parse("class A { }");
Optional<ClassOrInterfaceDeclaration> classA = compilationUnit.getClassByName("A");
Analyze Java code
Suppose you want to look for fields which are public and not static. This is pretty easy using JavaParser:
compilationUnit.findAll(FieldDeclaration.class).stream()
.filter(f -> f.isPublic() && !f.isStatic())
.forEach(f -> System.out.println("Check field at line " +
f.getRange().map(r -> r.begin.line).orElse(-1)));
Transform Java code
Maybe you want to ensure all abstract classes have a name starting with Abstract:
compilationUnit.findAll(ClassOrInterfaceDeclaration.class).stream()
.filter(c -> !c.isInterface()
&& c.isAbstract()
&& !c.getNameAsString().startsWith("Abstract"))
.forEach(c -> {
String oldName = c.getNameAsString();
String newName = "Abstract" + oldName;
System.out.println("Renaming class " + oldName + " into " + newName);
c.setName(newName);
});
Generate Java code
CompilationUnit compilationUnit = new CompilationUnit();
ClassOrInterfaceDeclaration myClass = compilationUnit
.addClass("MyClass")
.setPublic(true);
myClass.addField(int.class, "A_CONSTANT", PUBLIC, STATIC);
myClass.addField(String.class, "name", PRIVATE);
String code = myClass.toString();
Components of JavaParser
JavaParser is both the name of the set of tools and of the main component: the parser. In addition to JavaParser there is another component in the set of tools: JavaSymbolSolver.The parser is extra lightweight (no dependencies at all!) and can give you super fast an AST for your Java code. Sometimes an AST is not enough because you want:
- Understand to which element a certain name refer: a field? A variable?
- You need to know the type of a complex expression
- You want to get a list of all the interfaces implemented (also indirectly) by a certain class
License
JavaParser is available either under the terms of the LGPL License or the Apache License. You as the user are entitled to choose the terms under which adopt JavaParser.
For details about the LGPL License please refer to LICENSE.LGPL.
For details about the Apache License please refer to LICENSE.APACHE.